Update April '23: Updated code to support a broader range of use cases.
When creating complex Pardot Forms, fields can be made dependent on other fields. This allows to show or hide certain inputs if the user selects pre-defined values. However, this does not work for checkboxes.
The following script can be used to hide and show fields when the user checks a checkbox.
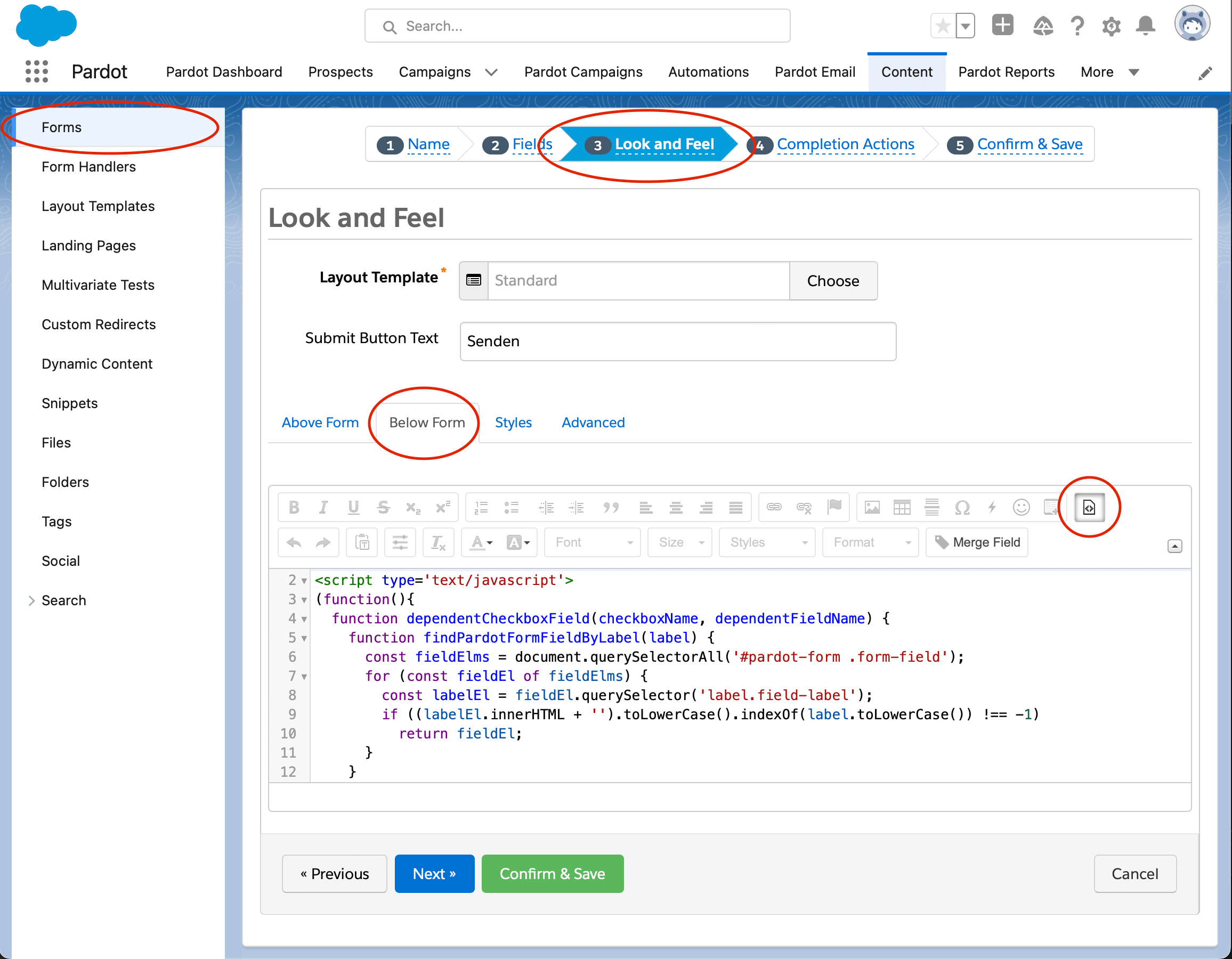
In Pardot, open your desired form and go to Step 3: Look and Feel -> Below Form -> HTML Mode (see screenshot) and insert an empty script tag:
<script type="text/javascript">
</script>
Between the script tags add the following javascript function:
function dependentCheckboxField(checkboxName, dependentFieldName) {
function normalizeString(str) {
return str.toLocaleLowerCase().replace(/\s/g, '').trim();
}
function findPardotFormFieldByLabel(label) {
for (const labelEl of document.querySelectorAll('#pardot-form label')) {
if (normalizeString(labelEl.innerHTML + '') === normalizeString(label))
return labelEl.parentElement;
}
}
const checkboxEl = findPardotFormFieldByLabel(checkboxName);
const dependentFieldEl = findPardotFormFieldByLabel(dependentFieldName);
function showDependentField(show) {
if (show) dependentFieldEl.style.removeProperty('display');
else dependentFieldEl.style.display = 'none';
}
checkboxEl.addEventListener('change', function (event) {
let checkedCount = parseInt(dependentFieldEl.dataset.checkedCount) || 0;
checkedCount = event.target.checked ? checkedCount + 1 : checkedCount - 1;
dependentFieldEl.dataset.checkedCount = checkedCount;
showDependentField(checkedCount > 0);
});
showDependentField(
checkboxEl.querySelector('input[type="checkbox"]').checked
);
}
After the javascript code (but before the closing script tag </script>
), you can now add dependent checkbox fields with:
dependentCheckboxField('Subscribe to Newsletter','E-Mail');
The first parameter is the name/label of the checkbox (e.g. Subscribe to Newsletter) and the second parameter is the name/label of the dependent field that should be visible when the checkbox is checked (e.g. E-Mail).
If you want to make multiple fields dependent on a single or even multiple checkboxes, you can just call the function multiple times with different parameters (don't forget the ;
at the end of each line). For example:
dependentCheckboxField('My first checkbox','E-Mail');
dependentCheckboxField('My first checkbox','Name');
dependentCheckboxField('My second checkbox','Address');
dependentCheckboxField('My second checkbox','E-Mail');
See full example on GitHub: https://gist.github.com/adroste/29772807dbb15b78671199e7a5da960d
Bonus: Hide Labels
The code above requires you to set a label for the checkbox and the dependent fields. In case you don't want to display some of the labels, you can hide them by adding the following javascript function between the script tags:
function hidePardotLabel(label) {
function normalizeString(str) {
return str.toLocaleLowerCase().replace(/\s/g, '').trim();
}
for (const labelEl of document.querySelectorAll('#pardot-form label')) {
if (normalizeString(labelEl.innerHTML + '') === normalizeString(label)) {
labelEl.style.display = 'none';
return;
}
}
}
Like above, you can hide the fields using:
hidePardotLabel('Subscribe to Newsletter');
If you want to hide multiple labels, you can also just call the function multiple times. For example:
hidePardotLabel('Label from checkbox 1');
hidePardotLabel('Label from checkbox 2');
hidePardotLabel('Label from dropdown');
hidePardotLabel('Label from input');